[ad name=”Google Adsense 468_60″]
Ron Fredericks writes: I am LectureMaker’s video platform technologist. So I thought I would demonstrate some of my sample code in building a simple website demo.
The demo consists of three pages that feature the following code
- PHP programming with OO and model-view-controller (MVC) architecture
- SQL file to create a new table and load some sample user data
- PHP sessions and OO MySQL Data Manager
- Use of CSS style sheet and tags for web page layout and forms management
- Use of JavaScript and jQuery functions and project library
- A RESTful API allowing users to move data between the three pages
Visit the running project’s website here:
www.lecturemaker.com/test/HP_login.php
Download a copy of the project’s source code from GitHub:
https://github.com/RonFredericks/PHP_Demo_Project
Overview
Build a 2-3 page website with a single page view concept with JavaScript mvc and REST service. The concept of the site is to choose your background. After login the user can select a file to upload and that becomes the background image of the landing page. Page 1 is login with at least 3 different users who can login. Page 2 is landing page which will show the image uploaded and page 3 is upload the background image you like. Page 2 and 3 should have a logout button.
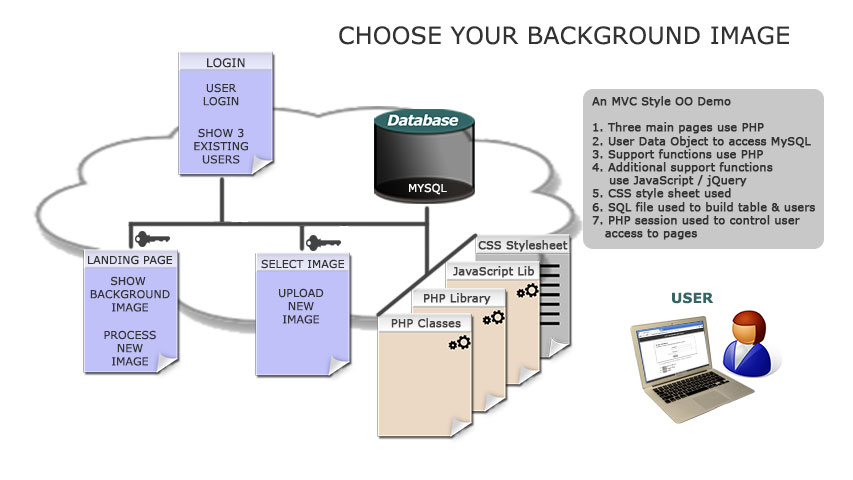
FAQ
What is OOP?
Object Oriented Programming, or OOP, invokes the use of classes to organize the data and structure of an application:
- Objects: instances of a class
- Inheritance: ability to pass characteristics and behaviors from a base class
- Access Modifiers: ability to protect data and methods
- Interfaces: a form of abstract class that acts as a model for creating a derived class
- PHP 5 Built-in Classes: Standard PHP Library (SPL), mysqli, PDO, SQLite, XML support, relfection, Iterator interface, magic methods, objects passed by reference
- Design Patterns: a reusable set of solutions that solve practical problems
What is MVC?
Model–View–Controller (MVC) is an architectural pattern used in software engineering:
- Model handles database logic. Code in the model connects to the database and provides an abstraction layer.
- Controller represents the business (application) logic i.e. all our ifs and else.
- View provides the presentation logic i.e our HTML/XML/JSON code.
What is REST?
Representational state transfer (REST) is a predominant web API design model for distributed systems. PHP examples include:
- Use FORM tag with GET or POST to send data to another web page.
- Use cURL to set the URL, create array of POST data, set options such as return XML data, and make request.
- Create a POST request by opening a TCP connection to the host using fsockopen(), then use fwrite() on the handler returned from fsockopen().
Design Methodology
See the figure above “Overview of the Project” where each web page is shown, along with the MySQL database supporting privileged access to the main project’s feature: to upload a new background image.
To start the project code I started with a basic development environment:
- WampServer: a Windows, Apache, MySQL, and PHP development environment. I chose this platform to run my test code because I can development the code directly within the Apache Localhost service without the delay of a repeated ftp style upload.
- Adobe’s DreamWeaver code editor: I use this code editing tool to highlight, format, and check my CSS, HTML, PHP, and JavaScript code. Because I save my files directly into the WampServer, the workflow is fairly efficient.
With this flexible coding environment I create three documents initially: the PHP login page, the CSS file, and a sql field definitions file using a tool called phpmyadmin hosted on my WampServer. I work back and forth between the login page, the CSS file, and the SQL definitions to get the look and feel I have visualized within my wireframe design. I add user fields into my SQL table to manage login storage. And as I start to see a pattern to the JavaScript and PHP support needs for this project: I prepare to add PHP classes, PHP support functions, and JavaScript code into new files as the code is development.
Create Login Page, CSS Stylesheet and SQL file
Create Landing Page
Create Image Upload Page
Create PHP Initialize File
Create Database Class
Create User Class
Create User Manager Class
////////////////////////////////// Create Login Page ///////////////////////
< ?php
// File: HP_login.php
// Purpose: Initialize and start PHP session
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
require_once './HP_assets/HP_initialize.php';
?>
< !doctype html>
Login Page
< ?php
// Insure that prior users are logged out of this web service
$um->logout();
// Initiate page div layout and display heading
PutHeader(HEADING);
// Initiate login
LoginForm("HP_uploadImage.php");
SelectValidUser();
// Complete page div layout and display footer
PutFooter(FOOTER);
exit();
?>
////////////////////////////////// Create Landing Page ///////////////////////
< ?php
// File: HP_landingPage.php
// Purpose: Initialize and start PHP session
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
require_once './HP_assets/HP_initialize.php';
?>
< !doctype html>
Landing Page
< ?php
PutHeader(HEADING);
if (!isset($user)) {
PutErrorMessage("You must log in to view this page: ".basename($_SERVER['PHP_SELF']));
Redirect('HP_login.php', 5);
exit();
}
?>
< ?php
PutErrorMessage(ProcessUploadForm());
PutFooter(FOOTER);
?>
////////////////////////////////// Create Image Upload Page ///////////////////////
< ?php
// File: HP_uploadImage.php
// Purpose: Initialize and start PHP session
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
require_once './HP_assets/HP_initialize.php';
?>
< !doctype html>
Upload Image
< ?php
// Attempt to process user login form $_POST
if (ProcessLoginForm($um)) {
// update user info on successful new login
$user = $um->getSession();
}
PutHeader(HEADING);
// Test for valid user, return to login page if user not valid
if (!isset($user)) {
PutErrorMessage("You must log in to view this page: ".basename($_SERVER['PHP_SELF']));
Redirect('HP_login.php', 5);
die();
}
?>
< ?php
// Initiate image upload form
GetImageForm("HP_landingPage.php");
DisplayValidImageTypes();
PutFooter(FOOTER);
?>
////////////////////////////////// Create PHP Initialize File ///////////////////////
< ?php
// File: HP_initialize.php
// Initialize PHP environment
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
define("HEADING", "Select Background Image Project");
define("FOOTER", "About Ron Fredericks");
define("IMAGE_DIR", "./HP_images/");
define("DEBUG", false); // set to true for more display messages
error_reporting(-1); // set to (-1) to display all errors, (0) for no errors and (E_ALL ^ E_NOTICE) for default production
global $validext; // define valid background image types as global
$validext = array(".gif"=>"GIF image", ".jpeg"=>"JPEG image", ".jpg"=>"JPG image", ".png"=>"PNG image");
// include the mySQL data management system
require_once './HP_assets/HP_UserManager.php';
require_once './HP_assets/HP_miscFunctions.php';
//
//session_start();
$um = new UserManager();
$user = $um->getSession();
////////////////////////////////// Create Database Class ///////////////////////
< ?php
// File: HP_Database.php
// Purpose: Define a class to MySQL database connection class
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
// References:
// http://forum.zonehacks.com/threads/10-PHP-User-Management-System-using-Object-Oriented-Programming-and-MySQL
// Object-Oriented PHP by Peter Lavin, No Starch Press, 2006
class Database {
private static $instances = 0; // make sure db connection is made only once, RDF
/**
* =============================================================
* Change these values to work with your mysql database settings
* =============================================================
*/
private $db_host = 'localhost';
private $db_user = 'xxx'; // fill in these values with your credentials
private $db_pass = 'xxx';
private $db_name = 'xxx'; // fill in your mySQL atabase name here
private $where = array();
private $variables = array();
private $link;
// see __call method below for implementaiton, RDF
private $functions = array(
'affected_rows' => 'mysql_affected_rows',
'client_encoding' => 'mysql_client_encoding',
'close' => 'mysql_close',
'connect' => 'mysql_connect',
'create_db' => 'mysql_create_db',
'data_seek' => 'mysql_data_seek',
'db_name' => 'mysql_db_name',
'db_query' => 'mysql_db_query',
'drop_db' => 'mysql_drop_db',
'errno' => 'mysql_errno',
'error' => 'mysql_error',
'escape_string' => 'mysql_escape_string',
'fetch_array' => 'mysql_fetch_array',
'fetch_assoc' => 'mysql_fetch_assoc',
'fetch_field' => 'mysql_fetch_field',
'fetch_lengths' => 'mysql_fetch_lengths',
'fetch_object' => 'mysql_fetch_object',
'fetch_row' => 'mysql_fetch_row',
'field_flags' => 'mysql_field_flags',
'field_len' => 'mysql_field_len',
'field_name' => 'mysql_field_name',
'field_seek' => 'mysql_field_seek',
'field_table' => 'mysql_field_table',
'field_type' => 'mysql_field_type',
'free_result' => 'mysql_free_result',
'get_client_info' => 'mysql_get_client_info',
'get_host_info' => 'mysql_get_host_info',
'get_proto_info' => 'mysql_get_proto_info',
'get_server_info' => 'mysql_get_server_info',
'info' => 'mysql_info',
'insert_id' => 'mysql_insert_id',
'list_dbs' => 'mysql_list_dbs',
'list_fields' => 'mysql_list_fields',
'list_processes' => 'mysql_list_processes',
'list_tables' => 'mysql_list_tables',
'num_fields' => 'mysql_num_fields',
'num_rows' => 'mysql_num_rows',
'pconnect' => 'mysql_pconnect',
'ping' => 'mysql_ping',
'query' => 'mysql_query',
'real_escape_string' => 'mysql_real_escape_string',
'result' => 'mysql_result',
'select_db' => 'mysql_select_db',
'set_charset' => 'mysql_set_charset',
'stat' => 'mysql_stat',
'tablename' => 'mysql_tablename',
'thread_id' => 'mysql_thread_id',
'unbuffered_query' => 'mysql_unbuffered_query'
);
function __construct()
{
if (Database::$instances == 0) {
$this->link = @$this->connect($this->db_host, $this->db_user, $this->db_pass); // catch and hide error messages using "@", RDF
if(!$this->link) {
die("Database class: ".mysql_error(). " Error no: ".mysql_errno()); // Include all possible error messages in display, RDF
}
$this->select_db($this->db_name);
Database::$instances = 1;
}
}
function __destruct()
{
if($this->link) {
Database::$instances == 0;
mysql_close($this->link);
unset($this->link); // Clear from memory, RDF
}
}
// Manage undeclared functions
public function __call($name, $arguments) {
if(isset($this->functions[$name])) {
return call_user_func_array($this->functions[$name], $arguments);
}
return FALSE;
}
}
////////////////////////////////// Create User Class ///////////////////////
< ?php
// File: HP_User.php
// Purpose: User class to manage undefined data members
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
// References:
// http://forum.zonehacks.com/threads/10-PHP-User-Management-System-using-Object-Oriented-Programming-and-MySQL
// Object-Oriented PHP by Peter Lavin, No Starch Press, 2006
class User
{
private $userdata = array();
public function checkPassword($pass)
{
if(isset($this->userdata['password']) && $this->userdata['password'] == md5($pass)) {
return true;
}
return false;
}
public function set($var, $value)
{
$this->userdata[$var] = $value;
}
public function get($var)
{
if(isset($this->userdata[$var])) {
return $this->userdata[$var];
}
return NULL;
}
}
////////////////////////////////// Create User Manager Class ///////////////////////
< ?php
// File: HP_UserManager.php
// Purpose: Create a class to manage user functions using a MySQL database
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/10/2013
// References:
// http://forum.zonehacks.com/threads/10-PHP-User-Management-System-using-Object-Oriented-Programming-and-MySQL
// Object-Oriented PHP by Peter Lavin, No Starch Press, 2006
// Include the User and Database class files
require_once "./HP_assets/HP_User.php";
require_once "./HP_assets/HP_Database.php";
class UserManager
{
private $db;
// Create an instance of the database class and store it into a private variable
public function UserManager()
{
$this->db = new Database();
}
public function createUser($username, $password, $email, $is_admin = FALSE)
{
if (isset($username) && isset($password) && isset($email)) { // Check for invalid function call, RDF
$stmt = sprintf("INSERT INTO users (`id`, `username`, `password`, `email`, `is_admin`) VALUES (NULL, '%s', '%s', '%s', '%d')",
$this->db->real_escape_string($username),
md5($this->db->real_escape_string($password)), // A md5 hash of the user's password will be stored in the database.
$this->db->real_escape_string($email), // always escape data from public before storing in database
$this->db->real_escape_string($is_admin));
$result = $this->db->query($stmt);
if ($result) return true;
}
return false;
}
public function updateUser($user)
{
// Normally I wouldn't store session data in the database, but
// it can be changed to track cookies if you plan to go that
// route.
$session = $user->get('session');
if (!$session) $session = 0;
$stmt = sprintf("UPDATE users SET `username` = '%s', `password` = '%s', `email` = '%s', `is_admin` = '%d', `session` = '%s' WHERE `id` = '%d'",
$this->db->real_escape_string($user->get('username')),
$this->db->real_escape_string($user->get('password')),
$this->db->real_escape_string($user->get('email')),
$this->db->real_escape_string($user->get('is_admin')),
$this->db->real_escape_string($session),
$this->db->real_escape_string($user->get('id')));
return $this->db->query($stmt);
}
public function deleteUser($user)
{
$userID = $this->db->real_escape_string($user->get('id'));
return $this->db->query("DELETE FROM users WHERE `id` = '$userID'");
}
// Get users from the database and return a user object by id or username
public function getUserByID($id)
{
if (isset($id)) { // Check for invalid function call, RDF
// get the user by id from database
$stmt = sprintf("SELECT * FROM users WHERE id = '%s'", $this->db->real_escape_string($id));
$result = $this->db->query($stmt);
if($result) {
$user = new User(); // create a new user object
$row = $this->db->fetch_assoc($result);
foreach($row as $key => $value) { // loop through user table values
$user->set($key, $value); // store them in the user object
}
return $user; // and return it
}
}
return NULL;
}
public function getUserByName($name)
{
if (isset($name)) { // Check for invalid function call, RDF
$stmt = sprintf("SELECT * FROM users WHERE username = '%s'", $this->db->real_escape_string($name));
$result = $this->db->query($stmt) or trigger_error(mysql_error()." ".$stmt);
if ($result && $this->db->num_rows($result) > 0) {
$user = new User();
$row = $this->db->fetch_assoc($result);
foreach($row as $key => $value) {
$user->set($key, $value);
}
return $user;
}
}
return NULL;
}
// Get user by name, check the password, updates session info in the database, and return the user object
public function login($username, $password)
{
if (isset($username) && isset($password)) { // Check for invalid function call, RDF
$user = $this->getUserByName($username);
if (isset($user) && $user->checkPassword($password)) {
// start PHP session, RDF
if(!isset($_SESSION)) session_start();
$_SESSION['zhuser'] = $user->get('username'); // I normally use these for cookies
$_SESSION['zhsess'] = md5($username.microtime()); // calculate md5 of username + current unix time
$user->set('session', $_SESSION['zhsess']); // set the session in user object
$this->updateUser($user); // update the user
return $user; // and return the user object if we're good
}
}
return NULL;
}
public function logout()
{
if (isset($_SESSION)) {
unset($_SESSION);
session_destroy();
}
}
// Check if a session exists and against what we have stored in the database, if they match we're good
public function getSession()
{
// start PHP session, RDF
if (!isset($_SESSION)) session_start();
if (isset($_SESSION['zhuser']) && isset($_SESSION['zhsess'])) {
$user = $this->getUserByName($_SESSION['zhuser']);
if (!$user) $this->logout();
if ($user->get('session') == $_SESSION['zhsess']) {
return $user;
}
}
return NULL;
}
}
Create PHP Functions Page
< ?php
// File: HP_miscFunctions.php
// Purpose: PHP Visual display and support functions
// Author: Ron Fredericks, LectureMaker LLC
// Last Updated: 7/11/2013
//////////////////////////////////////////////////////////////////
// Login Support Functions //
//////////////////////////////////////////////////////////////////
function LoginForm($url)
// Present a login form
{
// Reference: http://designikx.wordpress.com/2010/04/07/pure-css-div-based-form-design-form-layout/
$myArray[0] = array("test" => "", "message" => "Username must be entered");
?>
< ?php
}
function SelectValidUser()
// Present list of valid users for login
{
?>
Login List
Load one of these valid user credentials into login window
- Ron Fredericks
- Tommy Tuba
- Admin
< ?php
}
function ProcessLoginForm($um)
{
if (isset($_REQUEST['username']) && isset($_REQUEST['password']))
{
// Attempt to login
$user = $um->login($_REQUEST['username'], $_REQUEST['password']);
// Login failed, try again
if(!$user)
{
PutHeader(HEADING);
PutErrorMessage ("Invalid login, try selecting a user from the list...");
Redirect("HP_login.php", 5);
PutFooter(FOOTER);
} else {
// login was succesful
return true;
}
}
// login was either not successful or no login info was requested
return false;
}
//////////////////////////////////////////////////////////////////
// Upload Image Support Functions //
//////////////////////////////////////////////////////////////////
function GetImageForm($url)
// Present a login form
{
// Reference: http://designikx.wordpress.com/2010/04/07/pure-css-div-based-form-design-form-layout/
?>
< ?php
}
function DisplayValidImageTypes()
// Present list of valid file types for image upload
{
global $validext;
echo '';
echo 'Valid File Types
';
echo 'Choose a file to load with one of these valid extensions:
';
echo '';
foreach ($validext as $key => $value) {
echo "- $value [$key]
";
}
echo '
';
echo '';
}
function UploadFile($origin, $dest, $tmp_name)
// reference: function GetImageForm($url)
{
global $validext;
$origin = strtolower(basename($origin));
$fulldest = $dest.$origin;
$filename = $origin;
$fileext = (strpos($origin,'.')===false?'':'.'.substr(strrchr($origin, "."), 1));
$validflag = false;
foreach($validext as $ext=>$type) {
if ($fileext === $ext) {
$validflag = true;
break;
}
}
if (!$validflag) {
PutErrorMessage("Error: invalid file extension [$fileext]");
return false;
}
for ($i=1; file_exists($fulldest); $i++) {
$fileext = (strpos($origin,'.')===false?'':'.'.substr(strrchr($origin, "."), 1));
$filename = substr($origin, 0, strlen($origin)-strlen($fileext)).'['.$i.']'.$fileext;
$fulldest = $dest.$filename;
}
if (move_uploaded_file($tmp_name, $fulldest)) return $filename;
return false;
}
function DeleteImageFiles($directory, $days)
// delete files from a diectory,
// return number of files deleted,
// $directory must be: 1) a relative address and 2) not the same directory as the php file, for safety
{
$count = 0;
if (($days >= 0) && ($directory == IMAGE_DIR) && (dirname(__FILE__) != $directory) ) {
$files = glob($directory."*");
$seconds = $days * 24 * 60 * 60;
foreach($files as $file) {
$filemtime=filemtime ($file);
if(is_file($file) && time()-$filemtime>= $seconds) {
$count++;
unlink($file);
}
}
}
return $count;
}
function ProcessUploadForm()
// reference: function GetImageForm($url)
{
$msg= "";
if (isset($_POST['Submit']) && isset($_FILES["file"]["name"])) {
if (empty($_FILES["file"]["name"])) {
PutErrorMessage("You did not select an image for landing page, please try again...");
Redirect('HP_uploadImage.php', 5);
die();
}
// process file name requested on client's computer as a result of client's use of the 'browse' button
if ($_FILES["file"]["error"] > 0) {
$msg .= "Error: " . $_FILES["file"]["error"] . "
";
}
else {
// file found
$fileCnt = DeleteImageFiles(IMAGE_DIR, 0); // remove old images
if (DEBUG) {
$msg .= "$fileCnt prior images removed from " . IMAGE_DIR . " image directory
";
$msg .= "Upload: " . $_FILES["file"]["name"] . "
";
$msg .= "Type: " . $_FILES["file"]["type"] . "
";
$msg .= "temp: " . $_FILES["file"]["tmp_name"] . "
";
$msg .= "Size: " . round($_FILES["file"]["size"] / 1024, 2) . " Kb
";
}
$result = UploadFile($_FILES["file"]["name"], IMAGE_DIR, $_FILES["file"]["tmp_name"]);
if ($result) {
if (DEBUG) {
$msg .= "Result: " . IMAGE_DIR . $result."
";
}
?>
< ?php
}
else {
$msg .= "Upload was not successful
";
}
}
}
return $msg;
}
//////////////////////////////////////////////////////////////////
// Page Display Functions //
//////////////////////////////////////////////////////////////////
function PutHeader($heading, $left="", $right="")
// Initialize web page structure and display heading
{
echo "";
echo "$heading".GetButtons()."";
echo "";
}
function PutFooter($footer)
// Complete web page structure and display footer
{
echo "";
echo " ";
echo "";
?>
< ?php
}
function PutErrorMessage($msg)
// Manage display format for error messages
{
if (!empty($msg)) {
echo "$msg";
}
}
function Redirect($url, $waitSeconds = 0)
// Redirect to another web page
{
//header("Location: $url"); // Rewrite the header
echo "";
echo "
";
?>
< ?php
PutFooter(FOOTER);
die ();
}
function GetButtons()
// Dislplay user options as buttons when logged in
{
$msg="";
if (isset($_SESSION["zhuser"]) && !empty($_SESSION["zhuser"])) {
$msg .= "
by
Comments
Leave a Reply